Programming
Python
Programming
To provide participants with a solid foundation in Python programming
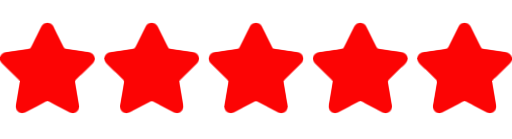
5
11 enrolled students
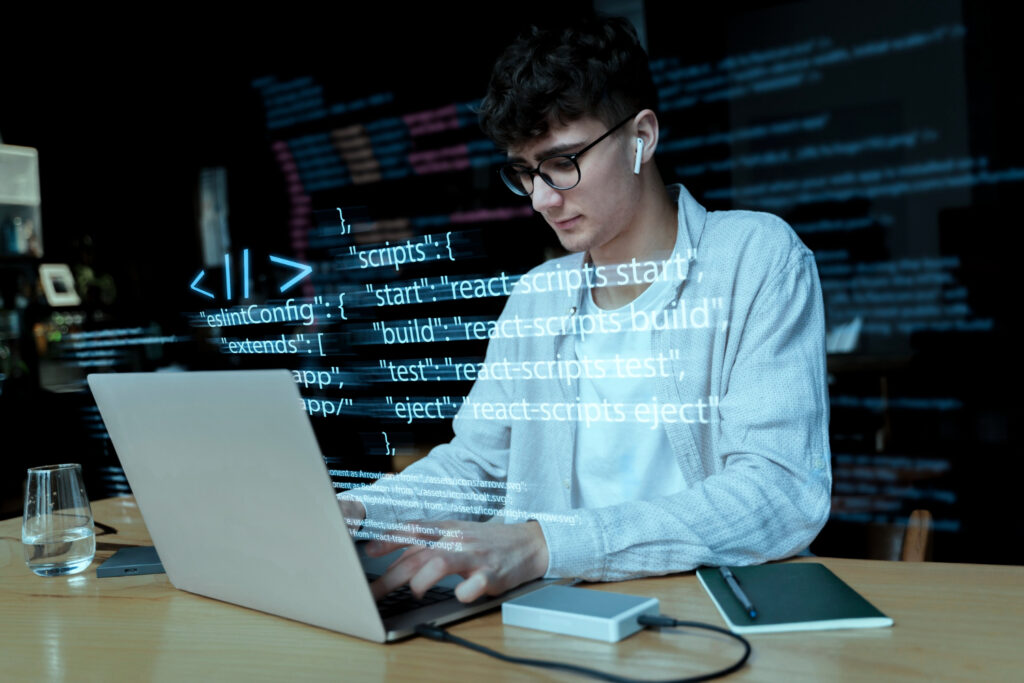
Objective
To provide participants with a solid foundation in Python programming, enabling them to write clean, efficient code, and apply core programming concepts in various real-world applications, including data manipulation, automation, and software development.
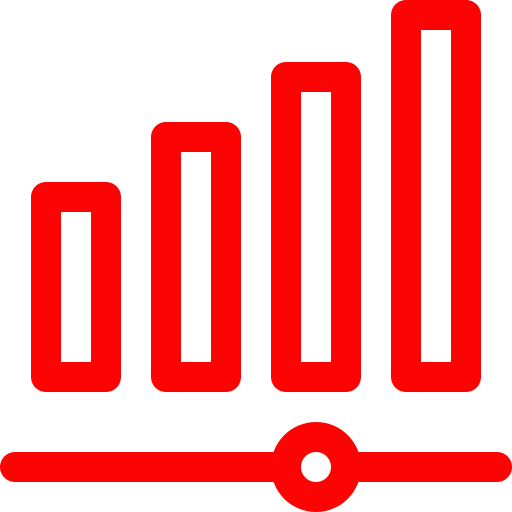
Basic To Advance
You will progress through this course from basics to advanced level.
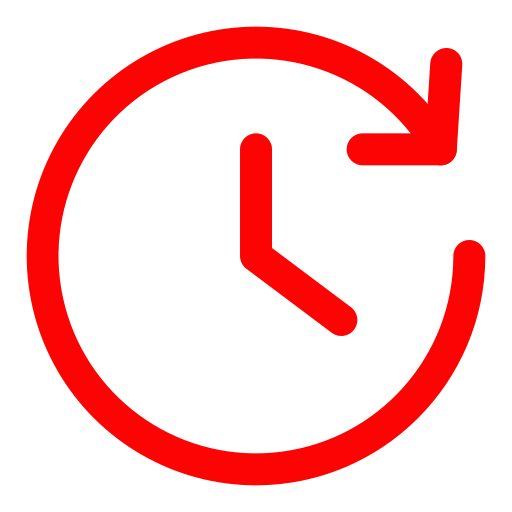
Duration
3 Months
Got questions?
Fill the form below and a Learning Advisor will get back to you.
Modules
Module 1: Introduction to Python
Topics:
- History and Applications of Python
- Setting Up the Environment (IDEs, Jupyter Notebooks)
- Basic Syntax, Keywords, and Structure
Hands on exercises:
- Starting to write code
Module 2: Data Types and Variables
Topics:
- Numbers, Strings, Lists, Tuples, and Dictionaries
- Type Conversion and Casting
- Variable Naming and Best Practices
Hands on exercises:
- Datatypes practice
Module 3: Operators and Expressions
Topics :
- Arithmetic, Comparison, Logical, and Bitwise Operators
- Operator Precedence and Associativity
- Working with Expressions
Hands on exercises:
- Arithmetic operations
Module 4: Control Structures
Topics :
- Conditional Statements (if, elif, else)
- Loops (for, while) and Loop Control (break, continue)
- Nested Loops and Conditionals
Hands on exercises:
- Conditional flows
- Iterating your program
Module 5: Functions and Modules
Topics :
- Defining and Calling Functions
- Function Arguments, Return Values, and Scope
- Lambda Functions
- Modules and the import Statement
Hands on exercises:
- Writing modular programs
Module 6: Data Structures
Topics :
- Lists, Tuples, Sets, and Dictionaries
- List Comprehensions and Dictionary Comprehensions
- Stack, Queue, and Linked List Basics
Hands on exercises:
- Using data structures to solve problems
Module 7: File Handling
Topics :
- Reading and Writing Files
- File Operations (open, close, read, write, append)
- Working with JSON and CSV Files
Hands on exercises:
- Reading data from files
Module 8: Error and Exception Handling
Topics :
try, except, finally Blocks
● Catching Specific Exceptions
● Raising Exceptions
Hands on exercises:
- Writing quality code
Module 9: Object-Oriented Programming (OOP)
Topics :
- Classes and Objects
- Inheritance, Encapsulation, and Polymorphism
- Dunder Methods and Operator Overloading
Hands on exercises:
- Object oriented programming
Module 10: Working with Libraries
Topics:
- math, random, and datetime Libraries
- os and sys for System Operations
- collections for Specialized Data Structures
Hands on exercises:
- Libraries
Module 11: Introduction to Data Handling and Visualization
Topics:
- Using pandas for Data Manipulation
- Basic Plotting with matplotlib and seaborn
Hands on exercises:
- Reading data to data frames
Module 12: Introduction to Functional and Recursive Programming
Topics:
- Map, Filter, and Reduce
- Recursive Functions and Use Cases
Hands on exercises:
- Map reduce
Module 13: Basic Web Scraping (Optional)
Topics:
- Introduction to BeautifulSoup and requests
- Parsing HTML and Extracting Data
Hands on exercises:
- Web Scraping
Module 14: Basic Networking and APIs
Topics:
- HTTP Requests with requests
- Working with APIs and JSON Data
Hands on exercises:
- Starting with APIs
Module 15: Debugging and Best Practices
Topics:
- Using Debugging Tools and Techniques
- Writing Clean, Readable, and Optimized Code
- Code Documentation and Commenting
Hands on exercises:
- Developing efficient programs
Module 16: Final Project
Developing a Capstone Project to Integrate Concepts
1. Personal Expense Tracker:
- Build a command-line or simple GUI application that allows users to track daily expenses, categorize spending, and generate monthly summaries. This project reinforces data structures, file handling, and basic data analysis.
2. Weather Data Dashboard:
- Create a dashboard that pulls real-time weather data from an API (e.g., OpenWeatherMap) and visualizes it using libraries like matplotlib or seaborn. Users can select their city and see the latest weather information, reinforcing skills in APIs, data handling, and visualization.
3. Text-Based Adventure Game:
- Develop an interactive, text-based game where users make choices that influence the outcome of the story. This project focuses on control structures, functions, and OOP principles.
4. Web Scraper for Job Listings:
- Design a web scraper that extracts job postings from popular job websites (e.g., LinkedIn, Indeed) based on keywords and location. Display results in a structured format and allow users to save them in CSV files. This project involves web scraping, file handling, and data parsing.
5. Inventory Management System:
- Build a basic inventory system for a small business to manage stock levels, record sales, and generate restocking alerts. This project reinforces file handling, OOP, and data structures.
These projects encourage hands-on application of Python skills, with room for creativity and integration of different course concepts. Each project can be expanded or simplified depending on the students’ proficiency level.
Frequently Asked Questions
1. Who is this course for?
This course is designed for beginners, intermediate learners, and professionals looking to enhance their Python programming skills. No prior programming experience is required.
2. Do I need any prior programming knowledge?
No, this course is beginner-friendly and assumes no prior programming experience.
Ready to Elevate Your Tech Career?
Join thousands of learners who have transformed their careers with CodeHub USA
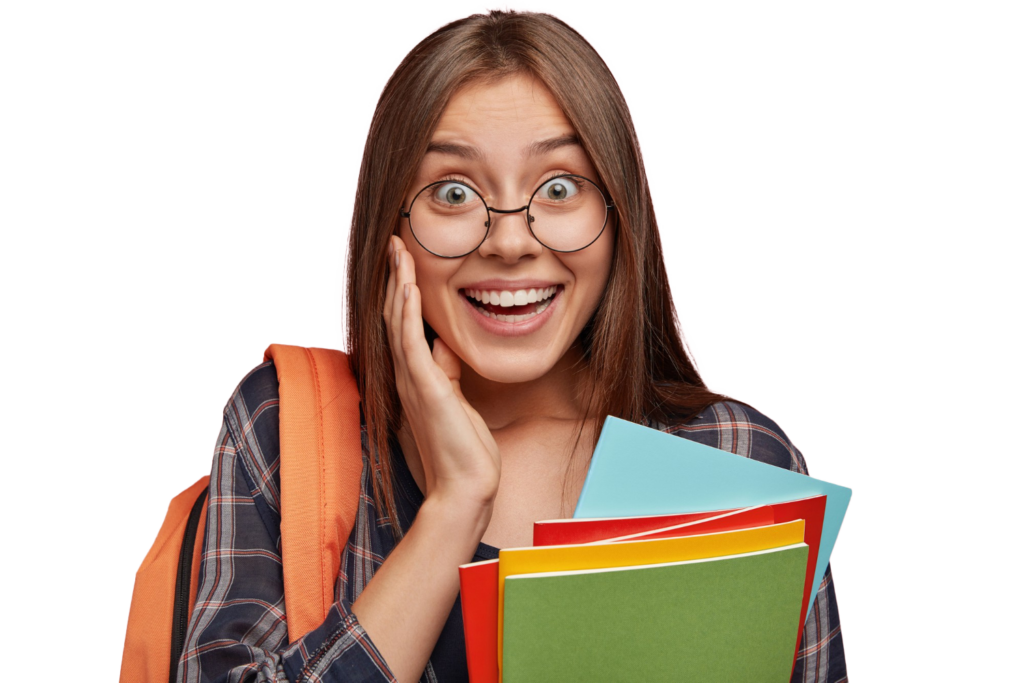